This will be a tutorial on how to debug a PHP application using xDebug and eclipse.
1. Get the eclipse for php from Eclipse for php
A side note, if you are on any *nix OS, this might require you to update your java to 1.7 and above. If that is the case, download latest Java JDK from Oracle (1.8 at the time of writing) and do:
which java
in most cases, this will show /usr/bin/java which is a symlink to the actual java binary.
Follow the symlink using
ls -la
That depending on the destro you have, would point to the link destination, there you can point to the java symlink to
ln -s /path/to/the/downloaded/jdk/bin/java
That should take care of the problem.
On windows, you need to updateh environment variable that you set for the JAVA_HOME. Googling on this shall give you the right direction.
2. Get xDebug on your system
Well, before this you might need the whole stack of apache, or any other php-approved webserver, and php itself. For that if you are on *nix you can use
sudo apt-get install lamp-server^
On windows, you can use wamp server and be done with it.
To install xdebug on *nix
sudo apt-get install php5-xdebug
Once you do that, restart your apache server
service apache2 restart
and do phpinfo(); you should be able to see the xdebug info there.
Now, pull your php.ini and add the following to it
[xdebug]
xdebug.remote_enable=on
xdebug.remote_handler=dbgp
xdebug.remote_port=9000
You can alternately add this info in the xdebug.ini if it it exists in the additional ini folder. This information would be available on the phpinfo();
Again restart your apache web server.
For testing purposes I have created a simple yet good php example on https://github.com/gullele/simple-php-calculator-example
Go to your eclipse Run>Run Configurations..
and do the following on respective tabs
For server tab:
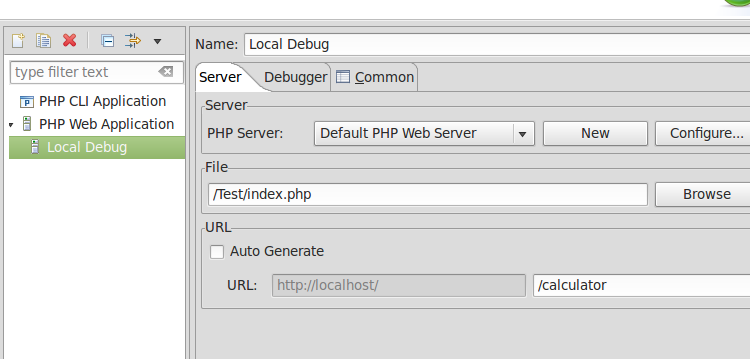
For Debugger tab.
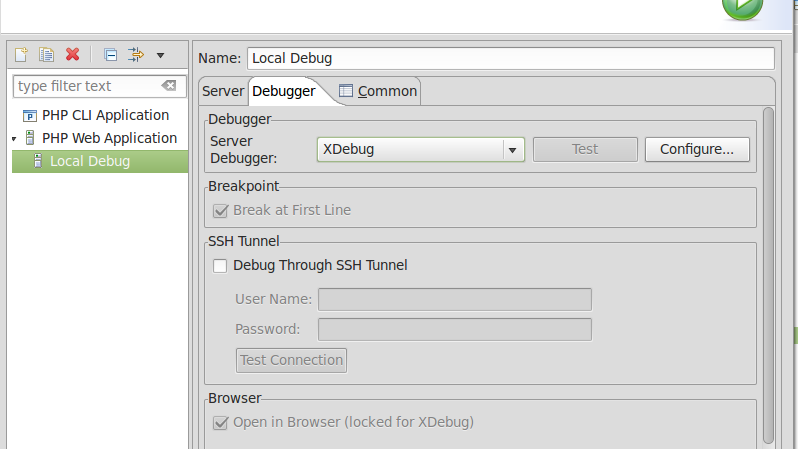
Now your eclipse is ready for debugging!
You can put a break point by double clicking on the area you would like the debugger to stop.
As you can see, I was able to see the value assigned on the $config variable that is was read from config.ini file.
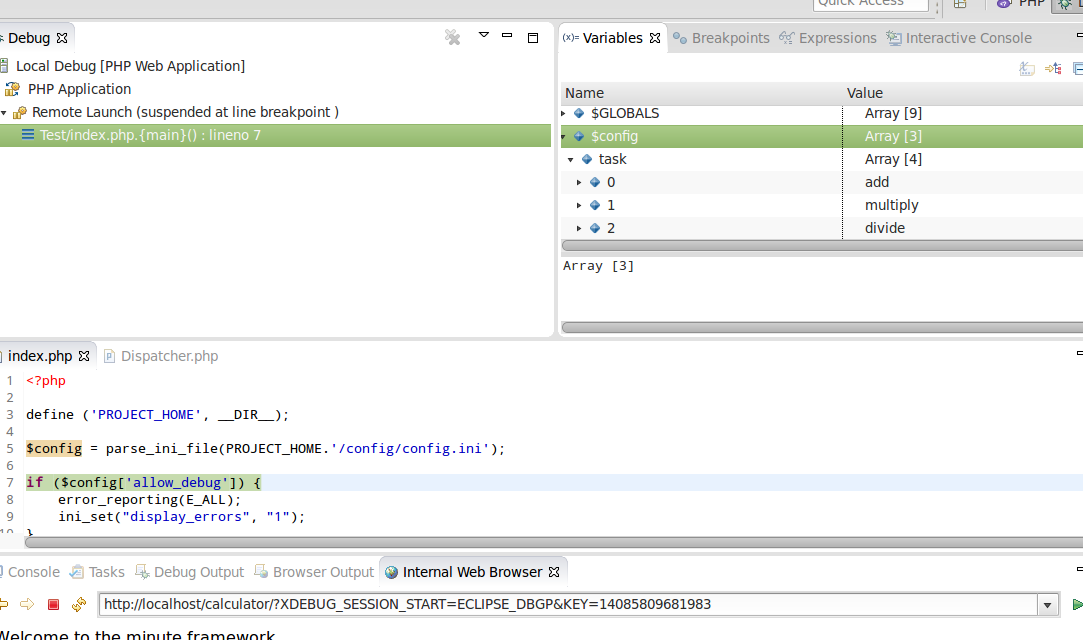