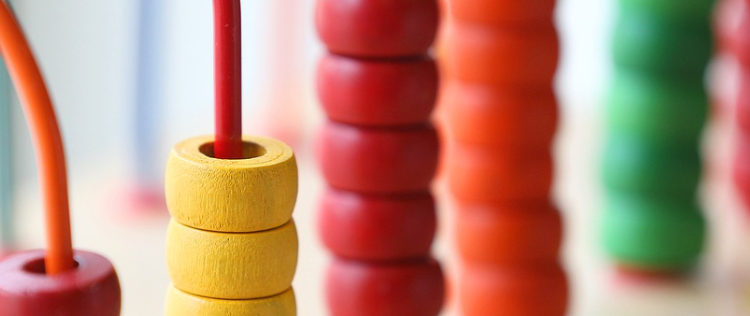
Find maximum occurring character from the given string
Max occurring character is the most frequent interview whiteboard question. Ok, there are so many of ways to handle this question and this is just one of them.
I have showed it with 6 characters as starting point and use those as reference. But you can extend that given object to hold as many characters as you want.
With the approach I am giving, the space complexity is constant as we can put all the characters in the keyboard to fit in the object..
Did i hear you saying, “How about unicodes??” You right, in that case it will be a bit out of control and doubt if it will be worth doing it in the object.
What other options are there to fit the character dictionary
How about we build it by going through string and also incrementing the value if we have it, and adding it if we don’t have it. object.hasOwnProperty(key)
will give us true or false if it has it so that way we will be efficient on space.
Regardless I want to proceed with this to leave room for improvement for anyone reading this. One can look at it and comeup with better space and time complexity
var str = "abbbcdddddddea";
var alpha = {a:0, b:0, c:0, d:0, e:0, f:0};
var max = 0;
var max_char = "";
for (ch of str) {
alpha[ch]++;
if (alpha[ch] > max) {
max = alpha[ch];
max_char = ch;
}
}
}
console.log (max_char + " has appeared " + max + " times");
You can run the above max character counting algorithm either right in chrome console or with javascript file that can be added in the HTML.
You may also be able to remove the max_char part and get it directly from whichever is the max. Just keep in mind when you do that, what will happen if we have two characters that are equally max in appearance?
Hope you have enjoyed the max character counting algorithm. If you have questions or if you have attempted the challenge, please share it here.
Find K Complementary numbers from array Java implementation
Check if there are three numbers a, b, c giving a total T from array A
binary tree problems with solution
Kadane’s algorithm in C – Dynamic Programming