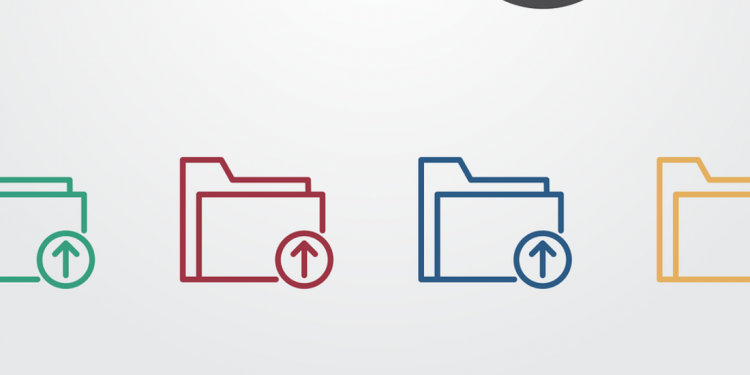
Spring upload multiple files with restful API
Spring upload multiple files with restful api is one of the most requires task. If you are working with spring, chances are high that you are working with file as well.
If you are doing registration, you might need to have multiple files to be uploaded to your server. So how to do spring upload multiple files is what I will be showing you.
Why multiple file upload in spring and spring boot
There are easier ways to upload single files using spring. But this will limit the user experience greatly. If you are allowing photos or documents to be uploaded, then you need to give an access for multiple file upload
What is needed for spring upload multiple files
I will be using jQuery for simplicity, but any Javascript framework or even plain javascript would work for this.
The front end for uploading multiple files using spring
<html><head>….
<form id=”form-upload”>
<input id=”file1″ type=”file” name=”file” />
<br />
<input id=”file2″ type=”file” name=”file” />
<br />
<button type=”button” class=”upload-multiple” value=”upload” />
</form>
The above snippet shows the required values for spring upload multiple files. Mind you in this example only two uploads are listed. But, it is basically unlimited.
That can be achieved using a simple javascript file to add another input for file.
Then lets look at how the jQuery to handle this looks like
//handle the button click event here.
$(document).ready(function() {
$(".upload-me").on("click", handleUpload); //on click, call handleUpload method
});
/**
* Handle actual handling of the files.
*/
function uploadFile() {
var url = "YOUR-BASE-URL-HERE"; //Like http://localhost:8081
var form = $('#form-upload')[0]; //get the form containing the files
var data = new FormData(form);
$.ajax({
url: url+"/api/files/upload",
type: "POST",
enctype: 'multipart/form-data',
data: data, //pass the form data
processData: false,
contentType: false,
success: function (data) {
console.log("Successfully uploaded " + data);
},
error: function (data) {
console.log("upload failed");
}
});
}
The above jQuery will take the files to be uploaded from the form and will post them to the given url to post the files.
Spring code to upload multiple files from the front end
package com.solutionladder.proofofconcept
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
/**
* A very simple and proof-of-concept only for uploading multiple files in
* spring/spring boot
*
* @author Kaleb Woldearegay
*
*/
@RestController
@RequestMapping(path = "/api/files")
@CrossOrigin(origins = { "*" })
public class FileUploadController {
/**
* The path to where the file will be uploaded
*/
private static String PATH_TO_UPLOAD = "/tmp/";
/**
* Check the file name on the form or curl has to be "file" that is what the
* method is looking for.
*
* @param files
* @return
*/
@PostMapping("/upload")
public String uploadFilez(@RequestParam("file") MultipartFile[] files) {
if (files.length == 0) {
return "Looks like there are no files uploaded";
}
try {
for (MultipartFile file : files) {
byte[] bytes = file.getBytes();
Path path = Paths.get(PATH_TO_UPLOAD + file.getOriginalFilename());
Files.write(path, bytes);
}
} catch (IOException e) {
e.printStackTrace();
}
return "Uploaded fine";
}
}
The above java code will handle the file upload. It will take the array of the files, and will be putting them to the hardcoded value it has been given.
Add batch/multiple posts to Facebook Page – Graph API
Spring Data error: getOutputStream() has already been called for this response
jQuery Select Option from Json
pass all the jars in classpath when compiling java
Get your filezilla password from your mac