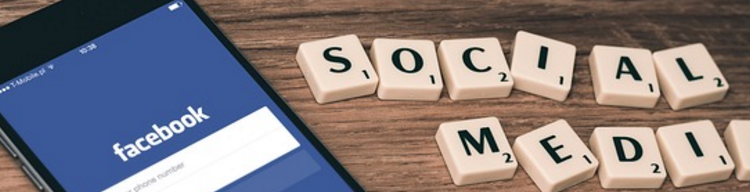
Post to Facebook From app – Using PHP
Post to Facebook programatically
You can post whatever you like from PHP to your or company’s page. Facebook has provided an SDK that makes life easier to post to the Facebook page.
This is ideal if you want to post to facebook, from cron job especially. Or on some event like right after the post in wordpress.
First thing first, you need to have a developer account on Facebook and you can do that easily and free on https://developers.facebook.com
Then you need to create an app. A single page or something like that would work. You will see how to create one when you are setting up your account for developer.
Once you have that, on the left menu, you will see dashboard. This contains important information about the app you will use to interact from your code.
The main information you need from this page are: APP ID, APP Secret and API Version
APP ID – This one will identify the app especially when you have more application under yoiur account.
APP Secret – well the name can tell about what it does.
APP Version – this is the default APP version you can access. The current version of facebook might be v5 right now. But if the APP Version says v2.10 then beyond this version you might not be able to access it.
Then download the SDK from here and put it, preferably, under your project or wherever you can access it.
Alternatively you can you git clone https://github.com/facebook/php-graph-sdk.git
Ok, now you have all the stuff you need.
Lets see the code.
/**
* Script for posting the videos to FB automatically.
* @author Kaleb
*/
define ('FACEBOOK_SDK_V5_SRC_DIR', __DIR__.'/php-graph-sdk/src/Facebook/');
require_once(__DIR__.'/php-graph-sdk/src/Facebook/autoload.php');
class FacebookPoster
{
private $facebook;
public function __construct(array $facebook_data)
{
$this->facebook = new Facebook\Facebook($facebook_data);
}
/**
* Post to facebook page using the given values.
*
*/
public function post(array $post_data, $token)
{
if (!empty($post_data)) {
try {
$post_response = $this->facebook->post('/me/feed', $post_data, $token);
} catch (Facebook\Exceptions\FacebookSDKException $ex) {
// $logger->log($ex->getMessage();
echo "Error occurred " . $ex->getMessage();
}
}
}
}
To use this snippet you will do the following:
$facebook_data = [
'app_id' => ‘APP_ID_GOES_HERE’,
'app_secret' => ‘APP_SECRET_GOES_HERE’,
'default_graph_version' => 'v2.2'
];
$facebook_poster = new FacebookPoster($facebook_data);
$post_data = [
'link' => 'YOUR LINK YOU WANT ON FACEBOOK',
'message' => 'YOUR MESSAGE YOU WANT ON THE POST'
];
$token =‘YOUR_TOKEN_GOES_HERE’;
//post it.
$facebook_poster->post($post_data, $token);
That concludes posting to facebook. Since this can post to facebook, all you have to do it to fetch the link and message from your posts or database and post it directly to your FB page.
connect to sftp from php
$_POST vs $HTTP_RAW_POST_DATA vs php://input and enctype
how to deploy symfony application to the production server
Deploying Symfony on Production Server
Run single phpunit test